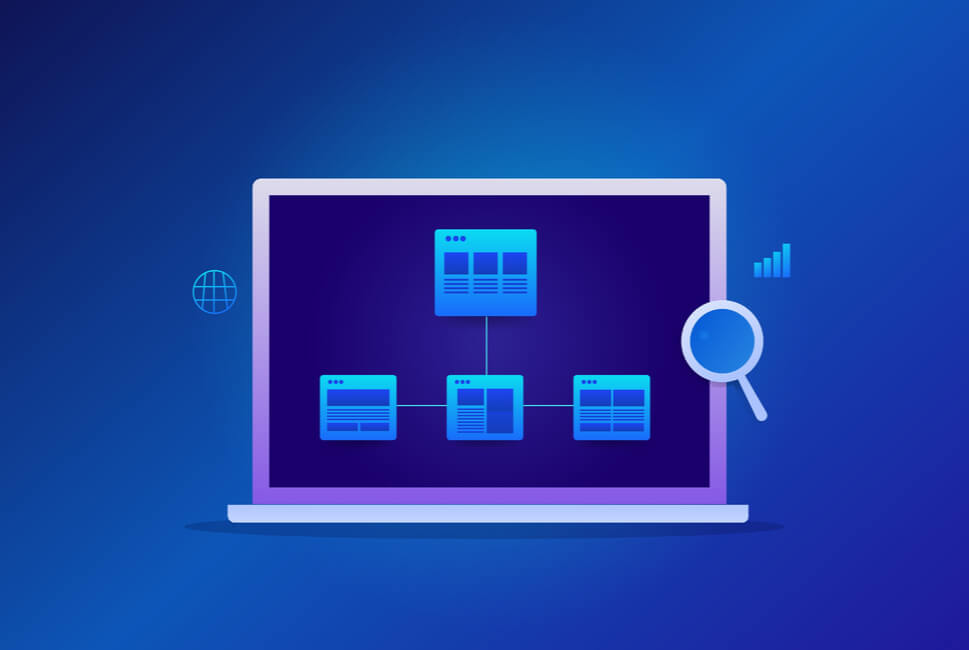
Published
Javier
How to create a sitemap in Laravel 9
Before deploying our Laravel project, we may need to create a sitemap to improve the SEO of our web page.
We can create a sitemap with Laravel in just three simple steps:
First, we create the route
We include the sitemap route in the file routes/web.php:
Route::get('/sitemap.xml', [SitemapXmlController::class, 'index']);
Second, we create the controller
We create the sitemap controller in the console:
php artisan make:controller SitemapXmlController
In the controller file app/Http/Controllers/SitemapXmlController.php we include:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Page;
class SitemapXmlController extends Controller
{
public function index() {
$pages = Page::where('publish', 1)->get();
return response()->view('sitemap', [
'pages' => $pages
])->header('Content-Type', 'text/xml');
}
}
Third, we create the view
We create the sitemap view in resources/views/sitemap.blade.php:
<?php echo '<?xml version="1.0" encoding="UTF-8"?>'; ?>
<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
@foreach ($pages as $page)
<url>
<loc>{{ url('/') }}/{{ $page->slug }}/</loc>
<lastmod>{{ $page->updated_at->tz('UTC')->toAtomString() }}</lastmod>
<changefreq>weekly</changefreq>
<priority>0.8</priority>
</url>
@endforeach
</urlset>
And that is all.
We can see our sitemap at https://ourwebpage/sitemap.xml